CyclicBarrier简单模拟订单对账流程
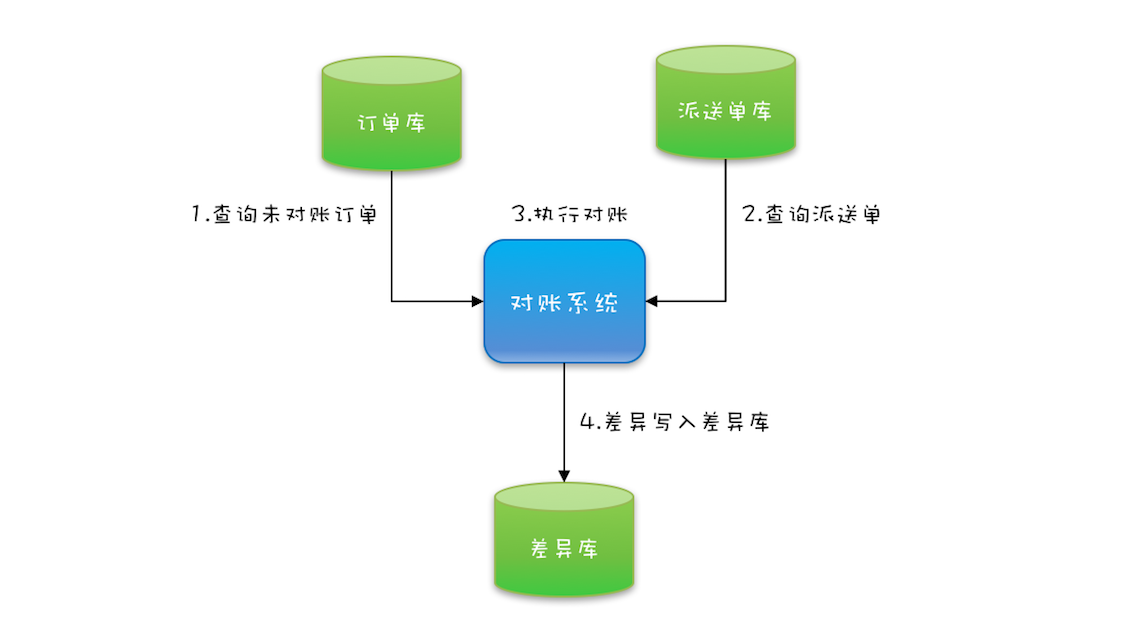
代码
public class CyclicBarrierDemo {
private static final int TICKET_NUM = 20;
private static final List<Integer> ORDER = Collections.synchronizedList(new ArrayList<>());
private static final List<Integer> SENDER = Collections.synchronizedList(new ArrayList<>());
private static final List<Integer> DIFFER_ORDER = Collections.synchronizedList(new ArrayList<>());
private static final List<Integer> DIFFER_SENDER = Collections.synchronizedList(new ArrayList<>());
private static final AtomicInteger ORDER_COUNT = new AtomicInteger(TICKET_NUM);
private static final AtomicInteger SENDER_COUNT = new AtomicInteger(TICKET_NUM);
private static final ThreadPoolExecutor POOL = (ThreadPoolExecutor) Executors.newFixedThreadPool(1);
private final CyclicBarrier notify = new CyclicBarrier(2, () -> {
POOL.execute(this::check);
});
static {
for (int i = 0; i < 20; i++) {
ORDER.add(i);
SENDER.add(new Random().nextInt(TICKET_NUM));
}
System.out.println("初始化订单数据: ");
System.out.println(ORDER);
System.out.println("初始化发货单数据: ");
System.out.println(SENDER);
}
public void check() {
int orderNum = DIFFER_ORDER.remove(0);
int senderNum = DIFFER_SENDER.remove(0);
if (orderNum == senderNum) {
System.out.println(orderNum + " == " + senderNum + " 订单和发货单无差异,无需处理...");
} else {
System.out.println(orderNum + " != " + senderNum + ", Order = " + orderNum + " 订单和发货单存在差异,需要调用对账方法...");
}
}
public void checkAll() {
new Thread(() -> {
while (ORDER_COUNT.get() != 0) {
try {
Thread.sleep((long) (Math.random() * 300));
DIFFER_ORDER.add(ORDER.get(TICKET_NUM - 1 - ORDER_COUNT.decrementAndGet()));
notify.await();
} catch (InterruptedException e) {
e.printStackTrace();
} catch (BrokenBarrierException e) {
e.printStackTrace();
}
}
}).start();
new Thread(() -> {
while (SENDER_COUNT.get() > 0) {
try {
Thread.sleep((long) (Math.random() * 300));
DIFFER_SENDER.add(SENDER.get(TICKET_NUM - 1 - SENDER_COUNT.decrementAndGet()));
notify.await();
} catch (InterruptedException e) {
e.printStackTrace();
} catch (BrokenBarrierException e) {
e.printStackTrace();
}
}
}).start();
}
public static void main(String[] args) {
CyclicBarrierDemo demo = new CyclicBarrierDemo();
demo.checkAll();
}
}
Run
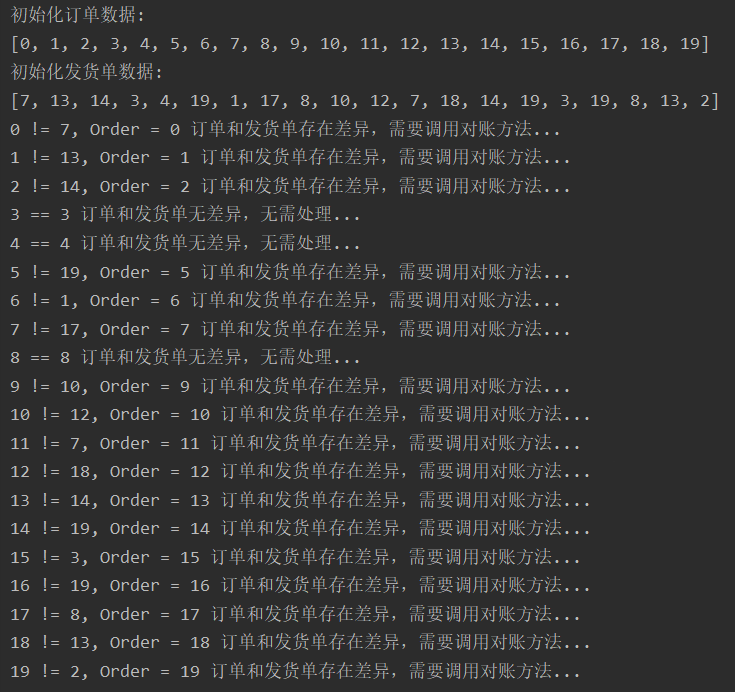